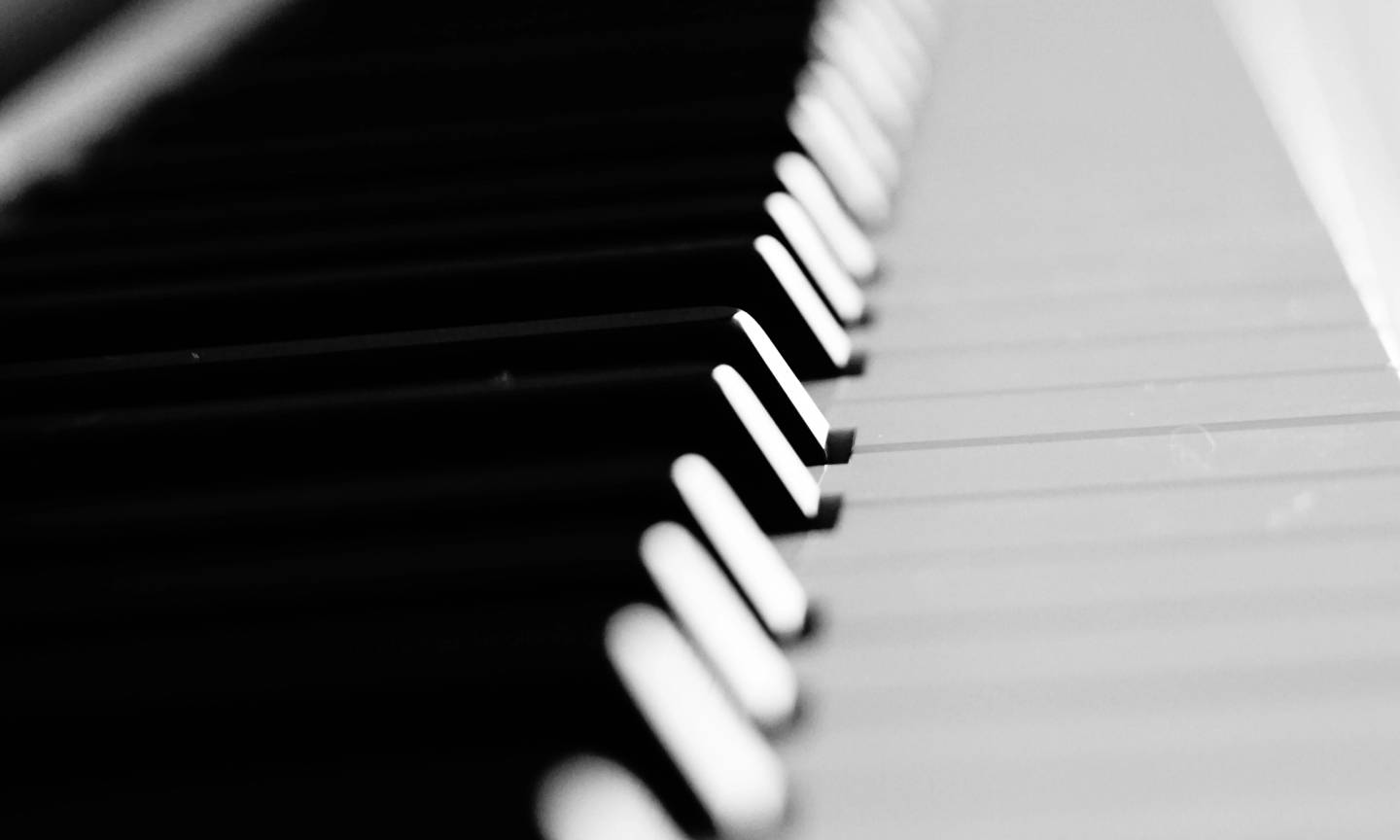
C# is a hugely popular, feature-rich and established programming language backed by one of the biggest tech companies in the world. Zig is a new, niche, high-performance, stripped-down programming language backed by a small charity and the open-source community. How do they stack up in terms of actual developer productivity and coding experience? Those depend on factors including the underlying architecture, memory management, syntax, community, high-level features and the speed of execution.
To explore those factors, I wrote the same test project in C# and in Zig, trying to stick to idiomatic syntax and patterns for each language. In this blog post, I’ll share the results of that process—including the strengths and weaknesses of each language, what fields they’re best suited to and how to maximize your productivity as a developer.
Architecture
To fully understand the other differences between C# and Zig, it’s important to understand the underlying architecture, as this affects syntax, compilation and execution times and, ultimately, developer experience.
C# is a bytecode-compiled language, similar to Java or Kotlin. This means there are two compilation steps: one to a platform-independent intermediate language at compile time, and then from the intermediate language (IL) to native code at run time. This is kind of a best-of-both worlds approach between interpreted/JIT languages like JavaScript and Python and compiled languages like Zig or C. Why? The IL (stored in a file with the extension .dll) is platform-independent (generally). So, the same file can be shared between Windows, Mac and Linux, as long as the .NET runtime is on the target machine. At the same time, the IL can be parsed and compiled faster than a text-based, human-written language like JavaScript or Python. It’s also faster to create IL from C# than to create an optimized native binary from Rust or Zig. Some dynamic features, like JIT optimizations and forms of dynamic typing, are also allowed. The downside of this approach is that runtime is generally slower than compiled programs because of the additional step of converting IL into native instructions.
Zig, on the other hand, compiles right from text to optimized native instructions. (Technically, Zig uses an intermediate representation too, but that IR is converted right to native instructions at compile time, it isn’t distributed.) So, compilation takes longer, there is no room for dynamic typing and you can only compile for one platform at a time. The benefits of this approach, however, are that it can be significantly faster to run (depending on the program) and the end user doesn’t need to install any extra software to be able to run it. Also, Zig makes it easier than almost any other compiled language to cross-compile (ie, compile something that will run on Windows from a Mac).
Technically, C# can be natively compiled (dotnet/aot) and Zig can be compiled to a cross-platform bytecode (WASM with WASI). But, these approaches are experimental and it’s less of a hassle to just use the default compilation strategy, at least for now.
So which approach is better? It depends entirely on what you’re using it for. C#’s two-step approach is great for GUI applications and video games, but Zig’s approach is best for when runtime performance is a major concern or you’re working with very limited resources. For example, if you’re working on code for a microcontroller or another bare-metal computer, Zig is a much better fit than C#.
Closely related to the underlying architecture and a huge factor for both developer experience and performance is memory management.
Memory Management
Memory management involves how a program allocates, uses and frees data that’s stored on the heap. That’s basically anything where the size isn’t known at compile time, like vectors, arrays, strings, classes & boxed data, files, etc. For most programs, this makes up the vast majority of memory usage.
C# is a garbage-collected (GC) language. This means any time you need to get memory on the heap, it happens transparently, references can be passed around wherever they need to go, and it’s freed once it’s not used anymore. This article explains in-depth how GC works in C#. Sounds great, eh? This approach to memory management is very much ‘don’t think about it’ for the average dev. So, the upside is clearly that it’s way easier and less tedious to write memory-safe code. The downside of this approach is you have little to no control over how things are allocated, where they’re allocated and when the GC runs (the garbage collector checks to see if anything on the heap can be freed). So, it’s much easier to get right, but if you’re short on memory, need custom allocation logic or real-time performance you might run into issues.
C# also provides an unsafe memory management interface where you can manually allocate, free and access memory. So, it’s there if you need it, but the majority of code you’ll be reading and writing will use the garbage-collected interface.
Zig only provides a manual memory interface. It actually provides one of the most low-level approaches of any modern language. In Zig, any function that might need to allocate or free something on the heap (so strings, vectors/lists, reading files, etc.) takes an Allocator
type as a parameter. And, anything that gets allocated on the heap has to be manually freed.
This approach is more finicky and tedious than C#’s, but Zig provides a couple features to help you out, like the defer
keyword to run a statement right before a function returns and a test allocator that tells you about leaks and other memory issues.
The main benefits of Zig’s approach are complete control over which allocator gets used and how, along with better performance and lower memory usage, if you do everything right. If you don’t set it up properly, it’s easy to introduce huge performance slowdowns, leaked allocations that drive up memory usage and even security issues. So, unless you need the extra control or speed that Zig gives, it’s better to stick with a more managed system (it doesn’t have to be GCed; even Rust has a more automatic approach than Zig). And, if you go with Zig, it’s vital to thoroughly test your program to detect leaks, double frees, use-after-frees and other memory issues.
Syntax
Zig and C# have fairly similar syntax: they’re both C-style languages. A lot comes down to personal preference. In terms of modules, I like Zig’s approach of imports and exports much more than C#’s default global namespace. I also like Zig’s imperative programming, function-based style more than C#’s object-oriented-everything-is-a-class style. Zig’s error-and-null handling keywords are also some of my favourite in any language. C# has a much bigger syntax than Zig—I personally find it hard to keep track of the differences between, say, private
, protected
, sealed
and internal
fields and methods, or what’s abstract
, what’s virtual
and what’s a record
.
C# has a very powerful syntax once you know it—they’ve been adding features for 20 years, so there’s not much it doesn’t have. Zig’s syntax, however, you can pick up in an afternoon if you’re familiar with a C-style language, but some solutions can be very verbose.
In general, that verbosity makes Zig more time-consuming to write and harder to understand at a glance, even though the syntax itself is simpler and smaller. However, a lot of that verbosity also comes down to a lack of high-level features.
High-level features
What exactly high-level features are can be a bit tricky to define, but I’d say it’s making common patterns shorter at the cost of control or performance. For example, to read a text file in C#:
using System.IO;
string fileContents = File.ReadAllText("path/to/file");
As opposed to Zig:
const std = @import("std");
var gpa = std.heap.GeneralPurposeAllocator(.{}){};
const allocator = gpa.allocator();
var file: []u8 = try std.fs.cwd().readFileAlloc(allocator, "path/to/file/relative/to/cwd", std.math.maxInt(isize));
defer allocator.free(file);
As you can see, Zig doesn’t even have a string type, it’s just a slice of bytes. And, it asks for information that C# just assumes, like which allocator to use, the maximum file size to accept and which directory to open. If you need that kind of control, this is great, but if not it ends up just being a pain.
In general, C# takes a more high-level approach to everything than Zig, with the result that it’s easier and less verbose to code the 99% of tasks that are the most common and then hard or impossible to code the 1% of least common tasks. Whereas, with Zig, everything is kind of inconvenient, but almost nothing is impossible to do.
Some valuable high-level features that C# has include asynchronous programming, attributes (they work like macros to add extra information to a function), the massive ‘standard library’ of ASP.NET and LINQ for working with queries and enumerables and operator overloading. These features make a lot of tasks easier in C# than in most other languages, especially one like Zig.
Related to abstractions built right into a language are the quality and variety of third-party packages.
Community
C# has been around for over 20 years and is continuously being promoted by one of the biggest tech companies in the world. Zig, on the other hand, was started in 2016 and is a primarily community-backed project led by a small nonprofit, the Zig Software Foundation. So, both languages have an active community, but C#’s is orders of magnitude bigger.
C#’s package manager, NuGet, has over 350,000 unique packages registered. Zig, on the other hand, doesn’t yet have a package manager. It’s planned for the language, but at the moment any dependencies have to be manually copied into a project.
C#’s community is a huge reason to use the language—with so many developers, any issues you run into have probably been seen before, and packages exist that abstract away many common tasks.
Zig’s community is growing, but the lack of both quantity and reliable quality of third-party packages makes some tasks more difficult. Also, issues you run into can be harder to diagnose and fix.
So, C# is easier to code in, has more high-level features and a bigger community than Zig. Then why would someone choose to program in Zig? Performance is a huge reason.
Performance
Comparing the performance of different programming languages is notoriously difficult. If you want to see a bunch of micro-benchmarks, check out https://programming-language-benchmarks.vercel.app/csharp-vs-zig.
I wrote a JSON parser using the same approach in both languages, and the Zig implementation ended up being an average of 90% faster (benchmarks for Zig and C#). While most programs won’t see that dramatic a speedup, in general well-written Zig will be faster than well-written C#, especially in areas where you need specialized memory allocation strategies.
Interestingly, even Zig compiled to a cross-platform bytecode (WASM) and then run in a runtime (Wasmtime) was still significantly faster than C#, so it’s not just about the architecture, the language itself plays a large role in performance.
However, poorly-written Zig will often be slower than C#, as C# is easier to write properly. So, if low memory usage and the highest performance are the priorities for your project and you’re willing to spend the time to write it properly, Zig might be a better fit. In general, however, C# will be more productive even with its slower execution.
Ultimately, it’s the same story in all the categories: C#’s architecture, memory management, syntax and features are all geared toward being productive as a developer at the cost of simplicity or performance. Zig, on the other hand, takes a pared-down, minimalistic approach to each of those factors that gives you a very fast language, but it’s harder to code higher-level applications in. Both languages are powerful and useful, but they’re intended uses are quite different. So, which should you use? For low-level, real-time or memory-constrained applications, Zig is a great alternative to C or Rust. But, for web servers, desktop applications or business logic, C# is a major player with good reason. By choosing the right tool for your job, you can continue to become more productive as a developer.